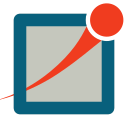 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_CSIMULATION_H
17 #define __OMNETPP_CSIMULATION_H
19 #include "simkerneldefs.h"
20 #include "simtime_t.h"
21 #include "ccomponent.h"
22 #include "ccontextswitcher.h"
23 #include "cexception.h"
36 class cFutureEventSet;
38 class cParsimPartition;
40 class cFingerprintCalculator;
44 class cUsageCollector;
46 SIM_API
extern cSoftOwner globalOwningContext;
70 static cEnvir *activeEnvir;
71 static cEnvir *staticEnvir;
77 int lastComponentId = 0;
81 cModule *systemModule =
nullptr;
84 ContextType contextType;
90 ContextType simulationStage;
95 bool trapOnNextEvent =
false;
97 bool parameterMutabilityCheck =
true;
100 cUsageCollector *usageCollector =
nullptr;
104 void checkActive() {
if (getActiveSimulation()!=
this)
throw cRuntimeError(
this, E_WRONGSIM);}
108 void setParameterMutabilityCheck(
bool b) {parameterMutabilityCheck = b;}
109 bool getParameterMutabilityCheck()
const {
return parameterMutabilityCheck;}
112 void parameterAccessed(
cPar *par);
135 virtual void forEachChild(
cVisitor *v)
override;
140 virtual std::string getFullPath()
const override;
167 static void setStaticEnvir(
cEnvir *env);
195 void deregisterComponent(
cComponent *component);
214 cModule *getModuleByPath(
const char *modulePath)
const;
228 cModule *findModuleByPath(
const char *modulePath)
const;
251 void setSystemModule(
cModule *module);
281 static int loadNedSourceFolder(
const char *folderName,
const char *excludedPackages=
"");
290 static void loadNedFile(
const char *nedFilename,
const char *expectedPackage=
nullptr,
bool isXML=
false);
302 static void loadNedText(
const char *name,
const char *nedText,
const char *expectedPackage=
nullptr,
bool isXML=
false);
310 static void doneLoadingNedFiles();
316 static std::string getNedPackageForFolder(
const char *folder);
350 void setSimulationTimeLimit(
simtime_t simTimeLimit);
362 void callInitialize();
374 void deleteNetwork();
467 void putBackEvent(
cEvent *event);
473 void executeEvent(
cEvent *event);
478 void callRefreshDisplay();
489 void transferToMain();
496 void insertEvent(
cEvent *event);
511 void setGlobalContext() {contextComponent=
nullptr; cOwnedObject::setOwningContext(&globalOwningContext);}
538 cModule *getContextModule()
const;
574 unsigned long getUniqueNumber();
581 void snapshot(
cObject *obj,
const char *label);
const typedef simtime_t & simtime_t_cref
Constant reference to a simtime_t.
Definition: simtime_t.h:48
Exception class.
Definition: cexception.h:49
This class represents modules in the simulation.
Definition: cmodule.h:48
simtime_t_cref getSimTime() const
Definition: csimulation.h:405
cComponent * getContext() const
Definition: csimulation.h:523
cObject is a lightweight class which serves as the root of the OMNeT++ class hierarchy....
Definition: cobject.h:92
cFingerprintCalculator * getFingerprintCalculator()
Definition: csimulation.h:587
int getSimulationStage() const
Definition: csimulation.h:385
void requestTrapOnNextEvent()
Definition: csimulation.h:552
This class defines the interface for fingerprint calculators.
Definition: cfingerprint.h:35
cEnvir * getEnvir()
Returns the environment object for the currently active simulation. This function never returns nullp...
Definition: csimulation.h:616
Utility class, to make it impossible to call the operator= and copy constructor of any class derived ...
Definition: cobject.h:415
Represents an event in the discrete event simulator.
Definition: cevent.h:46
Simulation manager class.
Definition: csimulation.h:64
void clearTrapOnNextEvent()
Definition: csimulation.h:557
bool isChannel() const
Definition: ccomponent.h:475
Abstract base class for the future event set (FES), a central data structure for discrete event simul...
Definition: cfutureeventset.h:32
Abstract class to encapsulate event scheduling.
Definition: cscheduler.h:47
Enables traversing the tree of (cObject-rooted) simulation objects.
Definition: cvisitor.h:56
Base class for channels.
Definition: cchannel.h:46
Abstract class for creating a module of a specific type.
Definition: ccomponenttype.h:206
simtime_t simTime()
Returns the current simulation time.
Definition: csimulation.h:601
bool isModule() const
Definition: ccomponent.h:470
int64_t-based, base-10 fixed-point simulation time.
Definition: simtime.h:66
cModule * getSystemModule() const
Definition: csimulation.h:256
cComponent * getComponent(int id) const
Definition: csimulation.h:234
bool isTrapOnNextEventRequested() const
Definition: csimulation.h:563
cScheduler * getScheduler() const
Definition: csimulation.h:332
int64_t eventnumber_t
Sequence number of events during the simulation. Events are numbered from one. (Event number zero is ...
Definition: simkerneldefs.h:78
cModule * getModule(int id) const
Definition: csimulation.h:240
cSimulation * getSimulation()
Returns the currently active simulation, or nullptr if there is none.
Definition: csimulation.h:608
int getLastComponentId() const
Definition: csimulation.h:200
Represents a module or channel parameter.
Definition: cpar.h:70
simtime_t_cref getWarmupPeriod() const
Definition: csimulation.h:422
ContextType getContextType() const
Definition: csimulation.h:532
void setWarmupPeriod(simtime_t t)
Definition: csimulation.h:427
Extends cObject with a name string. Also includes a "flags" member, with bits open for use by subclas...
Definition: cnamedobject.h:34
Common base for module and channel classes.
Definition: ccomponent.h:49
cFutureEventSet * getFES() const
Definition: csimulation.h:344
cChannel * getChannel(int id) const
Definition: csimulation.h:246
cSimpleModule * getActivityModule() const
Definition: csimulation.h:518
Thrown when the simulation kernel or other components detect a runtime error.
Definition: cexception.h:286
Base class for all simple module classes.
Definition: csimplemodule.h:202
cModuleType * getNetworkType() const
Definition: csimulation.h:391
static cEnvir * getActiveEnvir()
Definition: csimulation.h:154
cEnvir represents the "environment" or user interface of the simulation.
Definition: cenvir.h:75
static cEnvir * getStaticEnvir()
Definition: csimulation.h:172
static cSimulation * getActiveSimulation()
Definition: csimulation.h:148
void setSimTime(simtime_t time)
Definition: csimulation.h:398
eventnumber_t getEventNumber() const
Definition: csimulation.h:411
void setContextType(ContextType type)
Definition: csimulation.h:506
void setGlobalContext()
Definition: csimulation.h:511
cEnvir * getEnvir() const
Definition: csimulation.h:177