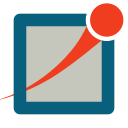 |
OMNeT++ NEDXML 6.1
Discrete Event Simulation Library
|
|
Go to the documentation of this file.
17 #ifndef __OMNETPP_NEDXML_NEDYYUTIL_H
18 #define __OMNETPP_NEDXML_NEDYYUTIL_H
21 #include "nedelements.h"
ParamElement * addParameter(ParseContext *np, ASTNode *params, YYLoc namepos)
PropertyElement * addComponentProperty(ParseContext *np, ASTNode *node, const char *name)
LiteralElement * createStringLiteral(ParseContext *np, YYLoc textpos)
void storeBannerComment(ParseContext *np, ASTNode *node, YYLoc tokenpos)
PropertyElement * storeComponentSourceCode(ParseContext *np, ASTNode *node, YYLoc tokenpos)
void storeInnerComments(ParseContext *np, ASTNode *node, YYLoc pos)
ASTNode * prependMinusSign(ParseContext *np, ASTNode *node)
void addExpression(ParseContext *np, ASTNode *elem, const char *attrname, YYLoc exprpos, ASTNode *expr)
void swapConnection(ASTNode *conn)
void storeRightComment(ParseContext *np, ASTNode *node, YYLoc tokenpos)
ASTNode * createNedElementWithTag(ParseContext *np, int tagcode, ASTNode *parent=nullptr)
Definition: astbuilder.h:25
PropertyElement * setIsNetworkProperty(ParseContext *np, ASTNode *node)
void storeFileComment(ParseContext *np, ASTNode *node)
PropertyElement * addProperty(ParseContext *np, ASTNode *node, const char *name)
LiteralElement * createPropertyValue(ParseContext *np, YYLoc textpos)
GateElement * addGate(ParseContext *np, ASTNode *gates, YYLoc namepos)
void storeBannerAndRightComments(ParseContext *np, ASTNode *node, YYLoc pos)
void addOptionalExpression(ParseContext *np, ASTNode *elem, const char *attrname, YYLoc exprpos, ASTNode *expr)
void storeTrailingComment(ParseContext *np, ASTNode *node, YYLoc tokenpos)
PropertyElement * storeSourceCode(ParseContext *np, ASTNode *node, YYLoc tokenpos)
LiteralElement * createQuantityLiteral(ParseContext *np, YYLoc textpos)
LiteralElement * createLiteral(ParseContext *np, int type, YYLoc valuepos, YYLoc textpos)
void addComment(ParseContext *np, ASTNode *node, const char *locId, const char *comment, const char *defaultValue)
ASTNode * getOrCreateNedElementWithTag(ParseContext *np, int tagcode, ASTNode *parent)