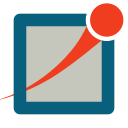 |
OMNeT++ NEDXML 6.1
Discrete Event Simulation Library
|
|
Go to the documentation of this file.
18 #ifndef __OMNETPP_NEDXML_NEDTYPEINFO_H
19 #define __OMNETPP_NEDXML_NEDTYPEINFO_H
24 #include "common/commonutil.h"
25 #include "nedelements.h"
30 class NedResourceCache;
46 enum Type {SIMPLE_MODULE, COMPOUND_MODULE, MODULEINTERFACE, CHANNEL, CHANNELINTERFACE};
57 bool resolved =
false;
58 bool resolving =
false;
84 void checkComplianceToInterface(
NedTypeInfo *interfaceDecl);
85 void collectLocalDeclarations();
98 virtual const char *getName()
const;
101 virtual const char *getFullName()
const;
104 virtual ASTNode *getTree()
const;
119 virtual bool isNetwork()
const;
124 virtual const char *getSourceFileName()
const;
130 virtual std::string getPackage()
const;
141 virtual const char *getEnclosingTypeName()
const;
146 virtual std::string str()
const;
151 virtual std::string getNedSource()
const;
165 virtual void resolve();
179 virtual const char *getExtendsName(
int k)
const;
195 virtual const char *getInterfaceName(
int k)
const;
202 virtual bool supportsInterface(
const char *qname);
210 virtual const char *getImplementationClassName()
const;
220 virtual std::string getPackageProperty(
const char *name)
const;
226 virtual std::string getCxxNamespace()
const;
236 TypesElement *getTypesElement()
const;
237 ParametersElement *getParametersElement()
const;
238 GatesElement *getGatesElement()
const;
239 SubmodulesElement *getSubmodulesElement()
const;
240 ConnectionsElement *getConnectionsElement()
const;
243 SubmoduleElement *getLocalSubmoduleElement(
const char *submoduleName)
const;
246 ConnectionElement *getLocalConnectionElement(
long id)
const;
249 SubmoduleElement *getSubmoduleElement(
const char *submoduleName)
const;
252 ConnectionElement *getConnectionElement(
long id)
const;
255 ParamElement *findLocalParamDecl(
const char *name)
const;
258 ParamElement *findParamDecl(
const char *name)
const;
261 GateElement *findLocalGateDecl(
const char *name)
const;
264 GateElement *findGateDecl(
const char *name)
const;
NameToElementMap localSubmoduleDecls
Definition: nedtypeinfo.h:79
Stores loaded NED files, and keeps track of components in them.
Definition: nedresourcecache.h:52
NameToElementMap localConnectionDecls
Definition: nedtypeinfo.h:80
NameToElementMap localParamDecls
Definition: nedtypeinfo.h:77
Type
Definition: nedtypeinfo.h:46
StringVector interfaceNames
Definition: nedtypeinfo.h:68
NedResourceCache * getResolver() const
Definition: nedtypeinfo.h:107
std::string implClassName
Definition: nedtypeinfo.h:73
#define NEDXML_API
Definition: nedxmldefs.h:31
ASTNode * tree
Definition: nedtypeinfo.h:55
virtual int numInterfaceNames() const
Definition: nedtypeinfo.h:188
Definition: astbuilder.h:25
std::vector< std::string > StringVector
Definition: nedtypeinfo.h:62
virtual int numExtendsNames() const
Definition: nedtypeinfo.h:172
NedResourceCache * resolver
Definition: nedtypeinfo.h:50
NameToElementMap localGateDecls
Definition: nedtypeinfo.h:78
Stores information on a NED type.
Definition: nedtypeinfo.h:43
virtual Type getType() const
Definition: nedtypeinfo.h:113
StringVector extendsNames
Definition: nedtypeinfo.h:67
std::map< std::string, ASTNode * > NameToElementMap
Definition: nedtypeinfo.h:63
virtual bool isResolved() const
Definition: nedtypeinfo.h:156
NameToElementMap allLocalDecls
Definition: nedtypeinfo.h:81
Type type
Definition: nedtypeinfo.h:52
NameToElementMap localInnerTypeDecls
Definition: nedtypeinfo.h:76
std::string qualifiedName
Definition: nedtypeinfo.h:53
std::string enclosingTypeName
Definition: nedtypeinfo.h:70
virtual bool isInnerType() const
Definition: nedtypeinfo.h:135
bool isInner
Definition: nedtypeinfo.h:54
void resolveIfNeeded() const
Definition: nedtypeinfo.h:88