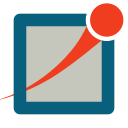 |
OMNeT++ NEDXML 6.1
Discrete Event Simulation Library
|
|
Go to the documentation of this file.
17 #ifndef __OMNETPP_NEDXML_MSGCOMPILER_H
18 #define __OMNETPP_NEDXML_MSGCOMPILER_H
28 #include "msgelements.h"
68 static constexpr
const char* ATT_NAME =
"name";
69 static const char *BUILTIN_DEFINITIONS;
72 void importBuiltinDefinitions();
73 void processBuiltinImport(
const char *txt,
const char *fname);
75 void processImport(ImportElement *importElem,
const std::string& currentDir);
76 std::string resolveImport(
const std::string& importName,
const std::string& currentDir);
77 void collectTypes(MsgFileElement *fileElement,
bool isImport);
78 void generateCode(MsgFileElement *fileElement);
79 std::string prefixWithNamespace(
const std::string& name,
const std::string& namespaceName);
80 void validateNamespaceName(
const std::string& namespaceName,
ASTNode *element);
97 void generate(MsgFileElement *fileElement,
const char *hFile,
const char *ccFile, StringSet& outImportedFiles);
102 void printPropertiesLatexDocu(std::ostream& out);
Options for MsgCompiler.
Definition: msgcompileroptions.h:32
Definition: msgtypetable.h:232
MsgTypeTable::Properties Properties
Definition: msgcompiler.h:50
Definition: msgtypetable.h:64
Definition: msgtypetable.h:153
MsgCodeGenerator codegen
Definition: msgcompiler.h:60
StringSet importsSeen
Definition: msgcompiler.h:64
Code generator part of the message compiler.
Definition: msgcodegenerator.h:40
static const char * getBuiltinDefinitions()
Definition: msgcompiler.h:107
#define NEDXML_API
Definition: nedxmldefs.h:31
MsgTypeTable::Property Property
Definition: msgcompiler.h:49
MsgTypeTable::EnumInfo EnumInfo
Definition: msgcompiler.h:54
Definition: astbuilder.h:25
Definition: msgtypetable.h:223
~MsgCompiler()
Definition: msgcompiler.h:91
Definition: msgtypetable.h:39
StringSet importedFiles
Definition: msgcompiler.h:65
MsgTypeTable::ClassInfo ClassInfo
Definition: msgcompiler.h:52
Definition: msgtypetable.h:44
MsgTypeTable::FieldInfo FieldInfo
Definition: msgcompiler.h:51
ErrorStore * errors
Definition: msgcompiler.h:63
std::set< std::string > StringSet
Definition: msgcompiler.h:47
MsgTypeTable::EnumItem EnumItem
Definition: msgcompiler.h:53
MsgTypeTable typeTable
Definition: msgcompiler.h:58
MsgCompilerOptions opts
Definition: msgcompiler.h:57
Part of the message compiler. Produces ClassInfo/EnumInfo objects from the ASTNode tree....
Definition: msganalyzer.h:41
MsgAnalyzer analyzer
Definition: msgcompiler.h:59
std::vector< std::string > StringVector
Definition: msgcompiler.h:48
Definition: errorstore.h:37
Generates C++ code from a MSG file object tree.
Definition: msgcompiler.h:44
Definition: msgtypetable.h:76