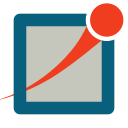 |
OMNeT++ NEDXML 6.1
Discrete Event Simulation Library
|
|
Go to the documentation of this file.
17 #ifndef __OMNETPP_NEDXML_MSGCODEGENERATOR_H
18 #define __OMNETPP_NEDXML_MSGCODEGENERATOR_H
29 #include "msgelements.h"
59 std::string prefixWithNamespace(
const std::string& name,
const std::string& namespaceName);
60 std::string makeFuncall(
const std::string& var,
bool isPointer,
const std::string& funcTemplate,
bool withIndex=
false,
const std::string& value=
"");
61 void generateOwnershipOp(
const FieldInfo& field,
const std::string& var,
const std::string& op);
63 void generateClassDecl(
const ClassInfo& classInfo,
const std::string& exportDef);
64 void generateClassImpl(
const ClassInfo& classInfo);
65 void generateStructDecl(
const ClassInfo& classInfo,
const std::string& exportDef);
66 void generateStructImpl(
const ClassInfo& classInfo);
67 void generateCplusplusBlock(std::ofstream& out,
const std::string& body);
68 void generateMethodCplusplusBlock(
const ClassInfo& classInfo,
const std::string& method);
69 void reportUnusedMethodCplusplusBlocks(
const ClassInfo& classInfo);
70 void generateDelegationForBaseClassFields(
const std::string& code);
74 void openFiles(
const char *hFile,
const char *ccFile);
77 void generateProlog(
const std::string& msgFileName,
const std::string& firstNamespace,
const std::string& exportDef);
78 void generateEpilog();
79 void generateClass(
const ClassInfo& classInfo,
const std::string& exportDef);
80 void generateStruct(
const ClassInfo& classInfo,
const std::string& exportDef);
81 void generateToAnyPtr(
const ClassInfo& a);
82 void generateFromAnyPtr(
const ClassInfo& a,
const std::string& exportDef);
83 void generateDescriptorClass(
const ClassInfo& a);
84 void generateEnum(
const EnumInfo& enumInfo);
85 void generateImport(
const std::string& importName);
86 void generateNamespaceBegin(
const std::string& namespaceName,
bool intoCcFile=
true);
87 void generateNamespaceEnd(
const std::string& namespaceName,
bool intoCcFile=
true);
88 void generateTypeAnnouncement(
const ClassInfo& classInfo);
89 std::string generatePreComment(
ASTNode *nedElement);
90 void generateHeaderCplusplusBlock(
const std::string& body);
91 void generateImplCplusplusBlock(
const std::string& body);
std::string headerGuard
Definition: msgcodegenerator.h:55
Definition: msgtypetable.h:232
Definition: msgtypetable.h:64
Definition: msgtypetable.h:153
std::vector< std::string > StringVector
Definition: msgcodegenerator.h:43
std::string hFilename
Definition: msgcodegenerator.h:51
Code generator part of the message compiler.
Definition: msgcodegenerator.h:40
std::ofstream ccStream
Definition: msgcodegenerator.h:54
MsgTypeTable::Properties Properties
Definition: msgcodegenerator.h:44
#define NEDXML_API
Definition: nedxmldefs.h:31
Definition: astbuilder.h:25
Definition: msgtypetable.h:223
MsgCodeGenerator(ErrorStore *errors)
Definition: msgcodegenerator.h:73
ErrorStore * errors
Definition: msgcodegenerator.h:56
MsgTypeTable::EnumItem EnumItem
Definition: msgcodegenerator.h:47
std::ofstream hStream
Definition: msgcodegenerator.h:53
std::string ccFilename
Definition: msgcodegenerator.h:52
MsgTypeTable::FieldInfo FieldInfo
Definition: msgcodegenerator.h:45
MsgTypeTable::ClassInfo ClassInfo
Definition: msgcodegenerator.h:46
Definition: errorstore.h:37
Definition: msgtypetable.h:76
MsgTypeTable::EnumInfo EnumInfo
Definition: msgcodegenerator.h:48