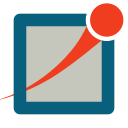 |
OMNeT++ NEDXML 6.1
Discrete Event Simulation Library
|
|
Go to the documentation of this file.
17 #ifndef __OMNETPP_NEDXML_MSGANALYZER_H
18 #define __OMNETPP_NEDXML_MSGANALYZER_H
28 #include "msgelements.h"
63 void analyzeClassOrStruct(
ClassInfo& classInfo,
const std::string& namespaceName);
64 void analyzeFields(
ClassInfo& classInfo,
const std::string& namespaceName);
65 void analyzeField(
ClassInfo& classInfo,
FieldInfo *field,
const std::string& namespaceName);
67 std::string prefixWithNamespace(
const std::string& name,
const std::string& namespaceName);
69 bool hasSuperclass(
ClassInfo& classInfo,
const std::string& superclassQName);
73 bool getPropertyAsBool(
const Properties& p,
const char *name,
bool defval);
74 std::string getProperty(
const Properties& p,
const char *name,
const std::string& defval = std::string());
75 std::string getMethodProperty(
const Properties& props,
const char *propName,
bool existingClass);
76 std::string decorateType(
const std::string& typeName,
bool isConst,
bool isPointer,
bool isRef);
77 std::string makeRelative(
const std::string& qname,
const std::string& namespaceName);
78 std::string lookupExistingClassName(
const std::string& name,
const std::string& contextNamespace, ClassInfo *contextClass=
nullptr);
79 void validateProperty(
const Property& property,
const char *usage);
84 ClassInfo extractClassInfo(
ASTNode *node,
const std::string& namespaceName,
bool isImported);
85 void ensureAnalyzed(ClassInfo& classInfo);
86 void ensureFieldsAnalyzed(ClassInfo& classInfo);
87 EnumInfo extractEnumDecl(EnumDeclElement *node,
const std::string& namespaceName);
88 EnumInfo extractEnumInfo(EnumElement *node,
const std::string& namespaceName);
89 ClassInfo extractClassInfoFromEnum(EnumElement *node,
const std::string& namespaceName,
bool isImported);
90 Property extractProperty(PropertyElement *propertyElem);
91 void validateFileProperty(
const Property& property);
92 void analyzeCplusplusBlockTarget(CplusplusElement *cppElem,
const std::string& currentNamespace);
95 static constexpr
const char* ATT_NAME =
"name";
96 static constexpr
const char* ATT_EXTENDS_NAME =
"extends-name";
98 static constexpr
const char* PROP_PROPERTY =
"property";
99 static constexpr
const char* PROP_ABSTRACT =
"abstract";
100 static constexpr
const char* PROP_ACTUALLY =
"actually";
101 static constexpr
const char* PROP_PRIMITIVE =
"primitive";
102 static constexpr
const char* PROP_OPAQUE =
"opaque";
103 static constexpr
const char* PROP_BYVALUE =
"byValue";
104 static constexpr
const char* PROP_SUPPORTSPTR =
"supportsPtr";
105 static constexpr
const char* PROP_SUBCLASSABLE =
"subclassable";
106 static constexpr
const char* PROP_POLYMORPHIC =
"polymorphic";
107 static constexpr
const char* PROP_CLASS =
"class";
108 static constexpr
const char* PROP_BASETYPE =
"baseType";
109 static constexpr
const char* PROP_DEFAULTVALUE =
"defaultValue";
110 static constexpr
const char* PROP_CPPTYPE =
"cppType";
111 static constexpr
const char* PROP_DATAMEMBERTYPE =
"datamemberType";
112 static constexpr
const char* PROP_ARGTYPE =
"argType";
113 static constexpr
const char* PROP_RETURNTYPE =
"returnType";
114 static constexpr
const char* PROP_TOSTRING =
"toString";
115 static constexpr
const char* PROP_FROMSTRING =
"fromString";
116 static constexpr
const char* PROP_TOVALUE =
"toValue";
117 static constexpr
const char* PROP_FROMVALUE =
"fromValue";
118 static constexpr
const char* PROP_GETTERCONVERSION =
"getterConversion";
119 static constexpr
const char* PROP_CLONE =
"clone";
120 static constexpr
const char* PROP_EXISTINGCLASS =
"existingClass";
121 static constexpr
const char* PROP_DESCRIPTOR =
"descriptor";
122 static constexpr
const char* PROP_CASTFUNCTION =
"castFunction";
123 static constexpr
const char* PROP_OMITGETVERB =
"omitGetVerb";
124 static constexpr
const char* PROP_FIELDNAMESUFFIX =
"fieldNameSuffix";
125 static constexpr
const char* PROP_BEFORECHANGE =
"beforeChange";
126 static constexpr
const char* PROP_IMPLEMENTS =
"implements";
127 static constexpr
const char* PROP_NOPACK =
"nopack";
128 static constexpr
const char* PROP_OWNED =
"owned";
129 static constexpr
const char* PROP_EDITABLE =
"editable";
130 static constexpr
const char* PROP_REPLACEABLE =
"replaceable";
131 static constexpr
const char* PROP_RESIZABLE =
"resizable";
132 static constexpr
const char* PROP_READONLY =
"readonly";
133 static constexpr
const char* PROP_OVERRIDEGETTER =
"overrideGetter";
134 static constexpr
const char* PROP_OVERRIDESETTER =
"overrideSetter";
135 static constexpr
const char* PROP_ENUM =
"enum";
136 static constexpr
const char* PROP_SIZETYPE =
"sizeType";
137 static constexpr
const char* PROP_SETTER =
"setter";
138 static constexpr
const char* PROP_GETTER =
"getter";
139 static constexpr
const char* PROP_GETTERFORUPDATE =
"getterForUpdate";
140 static constexpr
const char* PROP_SIZESETTER =
"sizeSetter";
141 static constexpr
const char* PROP_SIZEGETTER =
"sizeGetter";
142 static constexpr
const char* PROP_INSERTER =
"inserter";
143 static constexpr
const char* PROP_APPENDER =
"appender";
144 static constexpr
const char* PROP_ERASER =
"eraser";
145 static constexpr
const char* PROP_REMOVER =
"remover";
146 static constexpr
const char* PROP_ALLOWREPLACE =
"allowReplace";
147 static constexpr
const char* PROP_STR =
"str";
148 static constexpr
const char* PROP_CUSTOMIZE =
"customize";
149 static constexpr
const char* PROP_OVERWRITEPREVIOUSDEFINITION =
"overwritePreviousDefinition";
150 static constexpr
const char* PROP_CUSTOM =
"custom";
151 static constexpr
const char* PROP_CUSTOMIMPL =
"customImpl";
Options for MsgCompiler.
Definition: msgcompileroptions.h:32
Definition: msgtypetable.h:232
Definition: msgtypetable.h:64
static const StringSet RESERVED_WORDS
Definition: msganalyzer.h:54
Definition: msgtypetable.h:153
MsgTypeTable::EnumItem EnumItem
Definition: msganalyzer.h:50
MsgTypeTable::FieldInfo FieldInfo
Definition: msganalyzer.h:48
#define NEDXML_API
Definition: nedxmldefs.h:31
std::vector< std::string > StringVector
Definition: msganalyzer.h:45
bool hasProperty(const Properties &p, const char *name)
Definition: msganalyzer.h:72
Definition: astbuilder.h:25
Definition: msgtypetable.h:223
Definition: msgtypetable.h:39
Definition: msgtypetable.h:44
MsgTypeTable::Properties Properties
Definition: msganalyzer.h:47
std::set< std::string > StringSet
Definition: msganalyzer.h:44
MsgTypeTable::Property Property
Definition: msganalyzer.h:46
MsgTypeTable::EnumInfo EnumInfo
Definition: msganalyzer.h:51
MsgTypeTable::ClassInfo ClassInfo
Definition: msganalyzer.h:49
~MsgAnalyzer()
Definition: msganalyzer.h:83
const MsgCompilerOptions & opts
Definition: msganalyzer.h:59
MsgTypeTable * typeTable
Definition: msganalyzer.h:58
const Property * get(const std::string &name, const std::string &index="") const
Part of the message compiler. Produces ClassInfo/EnumInfo objects from the ASTNode tree....
Definition: msganalyzer.h:41
Definition: errorstore.h:37
Definition: msgtypetable.h:76
ErrorStore * errors
Definition: msganalyzer.h:57