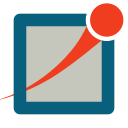 |
OMNeT++ NEDXML 6.1
Discrete Event Simulation Library
|
|
Go to the documentation of this file.
20 #ifndef __OMNETPP_NEDXML_ASTNODE_H
21 #define __OMNETPP_NEDXML_ASTNODE_H
25 #pragma warning(disable: 4996)
30 #include "common/pooledstring.h"
35 using omnetpp::common::opp_staticpooledstring;
69 opp_staticpooledstring
file;
73 FileLine(
const char *file,
int line=-1) : file(file), line(line) {}
74 bool empty()
const {
return file.empty();}
75 std::string
str()
const {
return empty() ?
"" : line == -1 ? file.str() : file.str() +
":" + std::to_string(line);}
92 opp_staticpooledstring directory;
102 static long numCreated;
103 static long numExisting;
106 static bool stringToBool(
const char *s);
107 static const char *boolToString(
bool b);
108 static int stringToEnum(
const char *s,
const char *vals[],
int nums[],
int n);
109 static const char *enumToString(
int b,
const char *vals[],
int nums[],
int n);
110 static void validateEnum(
int b,
const char *vals[],
int nums[],
int n);
135 virtual ASTNode *dup()
const = 0;
140 virtual ASTNode *dupTree()
const;
150 virtual const char *getTagName()
const = 0;
156 virtual int getTagCode()
const = 0;
161 virtual long getId()
const;
166 virtual void setId(
long id);
171 virtual FileLine getSourceLocation()
const;
176 virtual void setSourceLocation(
FileLine loc);
186 virtual const char *getSourceFileName()
const;
191 virtual int getSourceLineNumber()
const;
196 virtual const char *getSourceFileDirectory()
const;
208 virtual void setSourceRegion(
const SourceRegion& region);
220 virtual void applyDefaults();
226 virtual int getNumAttributes()
const = 0;
235 virtual const char *getAttributeName(
int k)
const = 0;
241 virtual int lookupAttribute(
const char *attr)
const;
251 virtual const char *getAttribute(
int k)
const = 0;
259 virtual const char *getAttribute(
const char *attr)
const;
269 virtual void setAttribute(
int k,
const char *value) = 0;
277 virtual void setAttribute(
const char *attr,
const char *value);
287 virtual const char *getAttributeDefault(
int k)
const = 0;
295 virtual const char *getAttributeDefault(
const char *attr)
const;
304 virtual ASTNode *getParent()
const;
310 virtual ASTNode *getFirstChild()
const;
316 virtual ASTNode *getLastChild()
const;
333 virtual ASTNode *getNextSibling()
const;
339 virtual ASTNode *getPrevSibling()
const;
346 virtual void appendChild(
ASTNode *node);
369 virtual ASTNode *getFirstChildWithTag(
int tagcode)
const;
385 virtual ASTNode *getNextSiblingWithTag(
int tagcode)
const;
391 virtual ASTNode *getPreviousSiblingWithTag(
int tagcode)
const;
396 virtual int getNumChildren()
const;
401 virtual int getNumChildrenWithTag(
int tagcode)
const;
411 ASTNode *getFirstChildWithAttribute(
int tagcode,
const char *attr,
const char *attrvalue=
nullptr);
417 ASTNode *getParentWithTag(
int tagcode);
441 virtual void setUserData(
UserData *data);
447 virtual UserData *getUserData()
const;
ASTNode * createElementWithTag(ParseContext *np, ASTNodeFactory *factory, int tagcode, ASTNode *parent=nullptr)
Subclass from this if you want to attach extra data to ASTNode objects.
Definition: astnode.h:42
int endLine
Definition: astnode.h:63
Stores a line:col..line:col region in a source file. Used for mapping ASTNodes back to the source cod...
Definition: astnode.h:59
ASTNode NedElement
Definition: astnode.h:451
int endColumn
Definition: astnode.h:64
UserData()
Definition: astnode.h:46
int startLine
Definition: astnode.h:61
#define NEDXML_API
Definition: nedxmldefs.h:31
Definition: astbuilder.h:25
void setSourceLocation(const char *fileName, int lineNumber)
Definition: astnode.h:181
opp_staticpooledstring file
Definition: astnode.h:69
virtual ~ASTNodeFactory()
Definition: astnode.h:462
static long getNumExisting()
Definition: astnode.h:432
FileLine(const char *file, int line=-1)
Definition: astnode.h:73
virtual ~UserData()
Definition: astnode.h:49
Base class for ASTNode factories.
Definition: astnode.h:459
FileLine()
Definition: astnode.h:72
bool empty() const
Definition: astnode.h:74
int startColumn
Definition: astnode.h:62
ASTNode MsgElement
Definition: astnode.h:452
static long getNumCreated()
Definition: astnode.h:426
std::string str() const
Definition: astnode.h:75