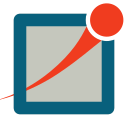 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
15 #ifndef __OMNETPP_STRINGUTIL_H
16 #define __OMNETPP_STRINGUTIL_H
23 #include "simkerneldefs.h"
60 return s ? strlen(s) : 0;
69 if (!s || !s[0])
return nullptr;
70 char *p =
new char[strlen(s)+1];
81 return strcpy(s1, s2 ? s2 :
"");
91 return s2 ? strcmp(s1,s2) : (*s1 ? 1 : 0);
93 return (s2 && *s2) ? -1 : 0;
99 SIM_API std::string
opp_trim(
const std::string& text);
111 _OPP_GNU_ATTRIBUTE(format(printf, 1, 2))
112 SIM_API std::
string opp_stringf(const
char *fmt, ...);
117 SIM_API std::
string opp_vstringf(const
char *fmt, va_list& args);
122 SIM_API std::
string opp_replacesubstring(const std::
string& text, const std::
string& substring, const std::
string& replacement,
bool replaceAll);
136 SIM_API std::vector<std::
string>
opp_split(const std::
string& text, const std::
string& separator);
143 SIM_API std::vector<std::
string>
opp_splitandtrim(const std::
string& text, const std::
string& separator);
158 SIM_API std::
string opp_substringbefore(const std::
string& str, const std::
string& substr);
163 SIM_API std::
string opp_substringafter(const std::
string& str, const std::
string& substr);
178 SIM_API std::
string opp_removestart(const std::
string& str, const std::
string& prefix);
183 SIM_API std::
string opp_removeend(const std::
string& str, const std::
string& end);
200 SIM_API const
char *
opp_strnistr(const
char *haystack, const
char *needle,
int n,
bool caseSensitive);
212 SIM_API std::
string opp_join(const
char *separator, const
char *s1, const
char *s2);
218 SIM_API std::
string opp_join(const
char *separator, const std::
string& s1, const std::
string& s2);
225 SIM_API std::
string opp_join(const
char **strings, const
char *separator,
bool skipEmpty=false,
char quoteChar=0);
232 SIM_API std::
string opp_join(const
char **strings,
int n, const
char *separator,
bool skipEmpty=false,
char quoteChar=0);
239 SIM_API std::
string opp_join(const std::vector<std::
string>& strings, const
char *separator,
bool skipEmpty=false,
char quoteChar=0);
244 SIM_API
char *
opp_itoa(
char *buf,
int d);
249 SIM_API
char *
opp_ltoa(
char *buf,
long d);
254 SIM_API
char *
opp_i64toa(
char *buf, int64_t d);
262 SIM_API
char *
opp_dtoa(
char *buf, const
char *format,
double d);
270 SIM_API
long opp_strtol(const
char *s,
char **endptr);
279 SIM_API
long opp_atol(const
char *s);
287 SIM_API
unsigned long opp_strtoul(const
char *s,
char **endptr);
296 SIM_API
unsigned long opp_atoul(const
char *s);
304 SIM_API
long long opp_strtoll(const
char *s,
char **endptr);
313 SIM_API
long long opp_atoll(const
char *s);
321 SIM_API
unsigned long long opp_strtoull(const
char *s,
char **endptr);
330 SIM_API
unsigned long long opp_atoull(const
char *s);
336 SIM_API
double opp_strtod(const
char *s,
char **endptr);
343 SIM_API
double opp_atof(const
char *s);
SIM_API std::string opp_removestart(const std::string &str, const std::string &prefix)
Remove the prefix if the s string starts with it, otherwise return the string unchanged.
SIM_API char * opp_ltoa(char *buf, long d)
Prints the d integer into the given buffer, then returns the buffer pointer.
SIM_API std::string opp_stringf(const char *fmt,...)
Create a string using printf-like formatting. Allocates storage dynamically.
SIM_API char * opp_strprettytrunc(char *dest, const char *src, unsigned maxlen)
Copies src string into dest, and if its length would exceed maxlen, it is truncated with an ellipsis....
SIM_API long opp_atol(const char *s)
Like the standard atol(), but throws opp_runtime_error if an overflow occurs during conversion,...
bool opp_isempty(const char *s)
Returns true if the string is nullptr or has zero length.
Definition: stringutil.h:35
SIM_API std::vector< std::string > opp_split(const std::string &text, const std::string &separator)
Split a string into parts separated by the given separator. If the input string is empty,...
SIM_API char * opp_dtoa(char *buf, const char *format, double d)
Prints the d double into the given buffer, then returns the buffer pointer. If d is finite,...
SIM_API double opp_atof(const char *s)
Like the standard atof(), but throws opp_runtime_error if an overflow occurs during conversion,...
SIM_API bool opp_isblank(const char *txt)
Returns true if the string only contains whitespace.
SIM_API double opp_strtod(const char *s, char **endptr)
Like the standard strtod(), but throws opp_runtime_error if an overflow occurs during conversion.
const char * opp_nulltoempty(const char *s)
Returns the pointer passed as argument unchanged, except that if it was nullptr, it returns a pointer...
Definition: stringutil.h:41
SIM_API char * opp_i64toa(char *buf, int64_t d)
Prints the d integer into the given buffer, then returns the buffer pointer.
SIM_API std::string opp_replacesubstring(const std::string &text, const std::string &substring, const std::string &replacement, bool replaceAll)
Performs find/replace within a string.
SIM_API long long opp_strtoll(const char *s, char **endptr)
Like the standard strtoll(), but throws opp_runtime_error if an overflow occurs during conversion....
char * opp_strdup(const char *s)
Duplicates the string, using new char[]. For nullptr and empty strings it returns nullptr.
Definition: stringutil.h:67
SIM_API unsigned long long opp_strtoull(const char *s, char **endptr)
Like the standard strtoull(), but throws opp_runtime_error if an overflow occurs during conversion....
SIM_API bool opp_stringbeginswith(const char *s, const char *prefix)
Returns true if the first string begins with the second string.
SIM_API std::string opp_substringafterlast(const std::string &str, const std::string &substr)
Returns the substring after the last occurrence of the given substring, or "".
SIM_API std::string opp_join(const std::vector< std::string > &strings, const char *separator, bool skipEmpty=false, char quoteChar=0)
Concatenate the strings passed in the vector, using the given separator, and putting each item betwee...
SIM_API char * opp_itoa(char *buf, int d)
Prints the d integer into the given buffer, then returns the buffer pointer.
const SIM_API char * opp_strnistr(const char *haystack, const char *needle, int n, bool caseSensitive)
Locates the first occurrence of the nul-terminated string needle in the string haystack,...
SIM_API std::string opp_substringbeforelast(const std::string &str, const std::string &substr)
Returns the substring up to the last occurrence of the given substring, or "".
SIM_API std::string opp_substringafter(const std::string &str, const std::string &substr)
Returns the substring after the first occurrence of the given substring, or "".
char * opp_strcpy(char *s1, const char *s2)
Same as the standard strcpy() function, except that nullptr in the second argument is treated as a po...
Definition: stringutil.h:79
SIM_API std::vector< std::string > opp_splitandtrim(const std::string &text, const std::string &separator)
Split a string into parts separated by the given separator, trimming each item of whitespace....
SIM_API unsigned long opp_atoul(const char *s)
Like the standard atol(), but for unsigned long, and throws opp_runtime_error if an overflow occurs d...
SIM_API std::string opp_substringbefore(const std::string &str, const std::string &substr)
Returns the substring up to the first occurrence of the given substring, or "".
int opp_strlen(const char *s)
Same as the standard strlen() function, except that does not crash on nullptr but returns 0.
Definition: stringutil.h:58
SIM_API long opp_strtol(const char *s, char **endptr)
Like the standard strtol(), but throws opp_runtime_error if an overflow occurs during conversion....
SIM_API long long opp_atoll(const char *s)
Like the standard atoll(), but throws opp_runtime_error if an overflow occurs during conversion,...
SIM_API std::string opp_strupper(const char *s)
Converts the string to upper case, and returns the result.
SIM_API int opp_strdictcmp(const char *s1, const char *s2)
Dictionary-compare two strings, the main difference from strcasecmp() being that integers embedded in...
SIM_API unsigned long long opp_atoull(const char *s)
Like the standard atoull(), but throws opp_runtime_error if an overflow occurs during conversion,...
SIM_API std::string opp_removeend(const std::string &str, const std::string &end)
Remove the given end string if the s string ends with it, otherwise return the string unchanged.
SIM_API std::string opp_vstringf(const char *fmt, va_list &args)
Create a string using printf-like formatting. Allocates storage dynamically.
SIM_API bool opp_stringendswith(const char *s, const char *ending)
Returns true if the first string ends in the second string.
SIM_API unsigned long opp_strtoul(const char *s, char **endptr)
Like the standard strtoul(), but throws opp_runtime_error if an overflow occurs during conversion....
SIM_API std::string opp_strlower(const char *s)
Converts the string to lower case, and returns the result.
int opp_strcmp(const char *s1, const char *s2)
Same as the standard strcmp() function, except that nullptr is treated exactly as an empty string (""...
Definition: stringutil.h:88
SIM_API std::string opp_trim(const std::string &text)
Removes any leading and trailing whitespace.
const char * opp_emptytodefault(const char *s, const char *defaultString)
Returns the pointer passed as argument unchanged, except that if it was empty, it returns the second ...
Definition: stringutil.h:47