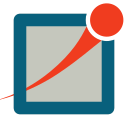 |
OMNeT++ API 6.2.0
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_RESULTRECORDERS_H
17 #define __OMNETPP_RESULTRECORDERS_H
20 #include "omnetpp/cresultrecorder.h"
44 VectorRecorder() {handle =
nullptr; lastTime = 0; lastValue = NAN;}
46 virtual simtime_t getLastWriteTime()
const {
return lastTime;}
47 virtual double getLastValue()
const {
return lastValue;}
48 virtual std::string str()
const override;
69 long getCount()
const {
return count;}
70 virtual std::string str()
const override;
99 double getLastValue()
const {
return lastValue;}
100 virtual std::string str()
const override;
128 double getSum()
const {
return sum;}
129 virtual std::string str()
const override;
140 bool timeWeighted =
false;
142 double lastValue = NAN;
144 double weightedSum = 0;
147 virtual void init(
Context *ctx)
override;
152 double getMean()
const;
153 virtual std::string str()
const override;
169 double getMin()
const {
return min;}
170 virtual std::string str()
const override;
186 double getMax()
const {
return max;}
187 virtual std::string str()
const override;
204 double getAverage()
const {
return sum/count;}
205 virtual std::string str()
const override;
215 double lastValue = NAN;
217 double weightedSum = 0;
224 double getTimeAverage()
const;
225 virtual std::string str()
const override;
237 double lastValue = NAN;
242 virtual void forEachChild(
cVisitor *v)
override;
247 virtual cStatistic *getStatistic()
const {
return statistic;}
248 virtual std::string str()
const override;
254 virtual void init(Context *ctx)
override;
257 class SIM_API HistogramRecorder :
public StatisticsRecorder
260 virtual void init(Context *ctx)
override;
263 class SIM_API TimeWeightedHistogramRecorder :
public StatisticsRecorder
266 virtual void init(Context *ctx)
override;
269 class SIM_API PSquareRecorder :
public StatisticsRecorder
272 virtual void init(Context *ctx)
override;
275 class SIM_API KSplitRecorder :
public StatisticsRecorder
278 virtual void init(Context *ctx)
override;
const typedef simtime_t & simtime_t_cref
Constant reference to a simtime_t.
Definition: simtime_t.h:48
Listener for recording the maximum of signal values. NaN values in the input are ignored.
Definition: resultrecorders.h:177
Listener for recording the arithmetic mean of signal values. NaN values in the input are ignored.
Definition: resultrecorders.h:194
Listener for recording a signal to an output vector.
Definition: resultrecorders.h:34
cObject is a lightweight class which serves as the root of the OMNeT++ class hierarchy....
Definition: cobject.h:92
Listener for recording signal values via a cStatistic. NaN values in the input are ignored,...
Definition: resultrecorders.h:233
Definition: cresultrecorder.h:82
Enables traversing the tree of (cObject-rooted) simulation objects.
Definition: cvisitor.h:56
Abstract base class for numeric result recorders.
Definition: cresultrecorder.h:128
Listener for recording the last non-NaN signal value.
Definition: resultrecorders.h:90
int64_t-based, base-10 fixed-point simulation time.
Definition: simtime.h:66
Listener for recording the sum of signal values. NaN values in the input are ignored.
Definition: resultrecorders.h:119
uint64_t uintval_t
Unsigned integer type which is guaranteed to be at least 64 bits wide. It is used throughout the libr...
Definition: simkerneldefs.h:109
Recorder that raises a runtime error if it sees a NaN in the input (and never records anything).
Definition: resultrecorders.h:107
Abstract base class for result recorders.
Definition: cresultrecorder.h:70
Listener for recording the (time-weighted or unweighted) mean of signal values. NaN values in the inp...
Definition: resultrecorders.h:137
Listener for recording the time average of signal values. NaN values in the input denote intervals to...
Definition: resultrecorders.h:212
#define SIMTIME_ZERO
Zero simulation time.
Definition: simtime_t.h:70
Listener for recording the count of signal values, including NaN and nullptr.
Definition: resultrecorders.h:54
int64_t intval_t
Signed integer type which is guaranteed to be at least 64 bits wide. It is used throughout the librar...
Definition: simkerneldefs.h:101
cStatistic is an abstract class for computing statistical properties of a random variable.
Definition: cstatistic.h:34
Base class for result filters.
Definition: cresultfilter.h:72
Listener for recording the count of signal values. Signal values do not need to be numeric to be coun...
Definition: resultrecorders.h:77
Listener for recording the minimum of signal values. NaN values in the input are ignored.
Definition: resultrecorders.h:160