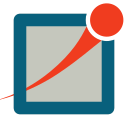 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_OPP_STRING_H
17 #define __OMNETPP_OPP_STRING_H
23 #include "simkerneldefs.h"
45 if (!s || !s[0])
return nullptr;
46 char *p =
new char[strlen(s)+1];
51 static int opp_strcmp(
const char *s1,
const char *s2) {
53 return s2 ? strcmp(s1,s2) : (*s1 ? 1 : 0);
55 return (s2 && *s2) ? -1 : 0;
72 opp_string(
const char *s,
int n) {buf =
new char[n+1]; strncpy(buf, s?s:
"", n); buf[n] =
'\0';}
97 const char *
c_str()
const {
return buf ? buf :
"";}
102 std::string
str()
const {
return buf ? buf :
"";}
107 bool empty()
const {
return !buf || !buf[0];}
119 int size()
const {
return buf ? strlen(buf) : 0;}
124 char *
reserve(
unsigned size) {
delete[] buf;buf=
new char[size];
return buf;}
189 inline std::ostream& operator<<(std::ostream& out,
const opp_string& s)
191 out << s.c_str();
return out;
char * buffer()
Definition: opp_string.h:114
~opp_string()
Definition: opp_string.h:92
const char * operator=(const char *s)
Definition: opp_string.h:130
Lightweight string vector, used internally in some parts of OMNeT++.
Definition: opp_string.h:203
opp_string & operator+=(const opp_string &s)
Definition: opp_string.h:165
opp_string operator+(const std::string &s)
Definition: opp_string.h:185
bool operator<(const opp_string &s) const
Definition: opp_string.h:145
opp_string & operator=(const std::string &s)
Definition: opp_string.h:140
std::string str() const
Definition: opp_string.h:102
Lightweight string class, used internally in some parts of OMNeT++.
Definition: opp_string.h:39
const char * c_str() const
Definition: opp_string.h:97
Lightweight string-to-string map, used internally in some parts of OMNeT++.
Definition: opp_string.h:219
opp_string(const char *s)
Definition: opp_string.h:67
char * opp_strdup(const char *s)
Duplicates the string, using new char[]. For nullptr and empty strings it returns nullptr.
Definition: stringutil.h:67
opp_string(const std::string &s)
Definition: opp_string.h:77
opp_string(const char *s, int n)
Definition: opp_string.h:72
opp_string & operator+=(const char *s)
Definition: opp_string.h:160
opp_string operator+(const char *s)
Definition: opp_string.h:175
opp_string(opp_string &&s)
Definition: opp_string.h:87
char * reserve(unsigned size)
Definition: opp_string.h:124
opp_string & operator=(const opp_string &s)
Definition: opp_string.h:135
opp_string(const opp_string &s)
Definition: opp_string.h:82
bool operator!=(const opp_string &s) const
Definition: opp_string.h:155
int size() const
Definition: opp_string.h:119
opp_string operator+(const opp_string &s)
Definition: opp_string.h:180
bool operator==(const opp_string &s) const
Definition: opp_string.h:150
opp_string()
Definition: opp_string.h:62
opp_string & operator+=(const std::string &s)
Definition: opp_string.h:170
bool empty() const
Definition: opp_string.h:107
int opp_strcmp(const char *s1, const char *s2)
Same as the standard strcmp() function, except that nullptr is treated exactly as an empty string (""...
Definition: stringutil.h:88