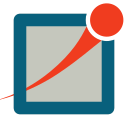 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_OPP_COMPONENT_PTR_H
17 #define __OMNETPP_OPP_COMPONENT_PTR_H
19 #include "ccomponent.h"
20 #include "cexception.h"
64 component->registerSelfPointer(ptr);
70 void deregisterPtr() {
72 dynamic_cast<cComponent*
>(ptr)->deregisterSelfPointer(ptr);
75 void checkNull()
const {
118 operator T *()
const {
return ptr;}
123 explicit operator bool()
const {
return ptr !=
nullptr;}
159 T *
get()
const {checkNull();
return ptr;}
T * get() const
Definition: opp_component_ptr.h:159
opp_component_ptr(const opp_component_ptr< T > &other)
Definition: opp_component_ptr.h:96
void operator=(T *newPtr)
Definition: opp_component_ptr.h:141
~opp_component_ptr()
Definition: opp_component_ptr.h:101
bool operator==(const T *ptr)
Definition: opp_component_ptr.h:129
Definition: opp_component_ptr.h:56
opp_component_ptr()
Definition: opp_component_ptr.h:84
void operator=(const opp_component_ptr< T > &ref)
Definition: opp_component_ptr.h:153
bool operator==(const opp_component_ptr< T > &other)
Definition: opp_component_ptr.h:135
T * getNullable() const
Definition: opp_component_ptr.h:164
T * operator->() const
Definition: opp_component_ptr.h:113
Common base for module and channel classes.
Definition: ccomponent.h:49
T & operator*() const
Definition: opp_component_ptr.h:107
Thrown when the simulation kernel or other components detect a runtime error.
Definition: cexception.h:286
opp_component_ptr(T *ptr)
Definition: opp_component_ptr.h:90
const SIM_API char * opp_typename(const std::type_info &t)
Returns the name of a C++ type, correcting the quirks of various compilers.