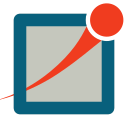 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_CTOPOLOGY_H
17 #define __OMNETPP_CTOPOLOGY_H
21 #include "cownedobject.h"
22 #include "csimulation.h"
32 #define INFINITY HUGE_VAL
72 std::vector<Link*> inLinks;
73 std::vector<Link*> outLinks;
83 Node(
int moduleId=-1) {this->moduleId=moduleId; weight=0; enabled=
true; dist=INFINITY; outPath=
nullptr;}
197 Link(
double weight=1) {srcNode=destNode=
nullptr; srcGateId=destGateId=-1; this->weight=weight; enabled=
true;}
333 virtual bool matches(
cModule *module) = 0;
337 std::vector<Node*> nodes;
338 Node *target =
nullptr;
341 static bool lessByModuleId(
Node *a,
Node *b) {
return (
unsigned int)a->moduleId < (
unsigned int)b->moduleId; }
342 static bool isModuleIdLess(Node *a,
int moduleId) {
return (
unsigned int)a->moduleId < (
unsigned int)moduleId; }
344 void unlinkFromSourceNode(Link *link);
345 void unlinkFromDestNode(Link *link);
348 virtual void parsimPack(cCommBuffer *)
const override {
throw cRuntimeError(
this, E_CANTPACK);}
349 virtual void parsimUnpack(cCommBuffer *)
override {
throw cRuntimeError(
this, E_CANTPACK);}
358 explicit cTopology(
const char *name=
nullptr);
363 cTopology(
const cTopology& topo);
368 virtual ~cTopology();
373 cTopology& operator=(
const cTopology& topo);
389 virtual std::string str()
const override;
407 virtual void extractFromNetwork(
bool (*selfunc)(
cModule *,
void *),
void *userdata=
nullptr);
412 virtual void extractFromNetwork(Predicate *predicate);
423 virtual void extractByModulePath(
const std::vector<std::string>& fullPathPatterns);
435 virtual void extractByNedTypeName(
const std::vector<std::string>& nedTypeNames);
455 virtual void extractByProperty(
const char *propertyName,
const char *value=
nullptr);
463 virtual void extractByParameter(
const char *paramName,
const char *paramValue=
nullptr);
468 virtual void clear();
478 virtual int addNode(Node *node);
484 virtual void deleteNode(Node *node);
491 virtual void addLink(Link *link, Node *srcNode, Node *destNode);
500 virtual void addLink(Link *link,
cGate *srcGate,
cGate *destGate);
506 virtual void deleteLink(Link *link);
526 virtual Node *getNode(
int i)
const;
535 virtual Node *getNodeFor(
cModule *mod)
const;
550 virtual void calculateUnweightedSingleShortestPathsTo(Node *target);
557 virtual void calculateWeightedSingleShortestPathsTo(Node *target);
This class represents modules in the simulation.
Definition: cmodule.h:48
virtual Link * createLink()
Definition: ctopology.h:575
int getNumOutLinks() const
Definition: ctopology.h:146
int getNumPaths() const
Definition: ctopology.h:166
Supporting class for cTopology.
Definition: ctopology.h:286
int getRemoteGateId() const
Definition: ctopology.h:305
Supporting class for cTopology.
Definition: ctopology.h:240
cGate * getRemoteGate() const
Definition: ctopology.h:315
bool isEnabled() const
Definition: ctopology.h:216
double getWeight() const
Definition: ctopology.h:103
int getNumInLinks() const
Definition: ctopology.h:136
cModule * getModule() const
Definition: ctopology.h:97
Supporting class for cTopology, represents a node in the graph.
Definition: ctopology.h:64
virtual int getNumNodes() const
Definition: ctopology.h:520
LinkOut * getPath(int) const
Definition: ctopology.h:173
void enable()
Definition: ctopology.h:222
virtual cTopology * dup() const override
Definition: ctopology.h:383
cModule * getModule(int id) const
Definition: csimulation.h:240
int getRemoteGateId() const
Definition: ctopology.h:259
cSimulation * getSimulation()
Returns the currently active simulation, or nullptr if there is none.
Definition: csimulation.h:608
Represents a module gate.
Definition: cgate.h:62
int getLocalGateId() const
Definition: ctopology.h:310
void setWeight(double d)
Definition: ctopology.h:210
void disable()
Definition: ctopology.h:127
Node(int moduleId=-1)
Definition: ctopology.h:83
Node * getRemoteNode() const
Definition: ctopology.h:295
void enable()
Definition: ctopology.h:121
virtual Node * createNode(cModule *module)
Definition: ctopology.h:570
int getId() const
Definition: ccomponent.h:433
cGate * getLocalGate() const
Definition: ctopology.h:320
void disable()
Definition: ctopology.h:228
virtual Node * getTargetNode() const
Definition: ctopology.h:563
Base class for selector objects used in extract...() methods of cTopology.
Definition: ctopology.h:329
double getDistanceToTarget() const
Definition: ctopology.h:160
double getWeight() const
Definition: ctopology.h:204
Link(double weight=1)
Definition: ctopology.h:197
Supporting class for cTopology, represents a link in the graph.
Definition: ctopology.h:181
Routing support. The cTopology class was designed primarily to support routing in telecommunication o...
Definition: ctopology.h:54
Node * getRemoteNode() const
Definition: ctopology.h:249
Node * getLocalNode() const
Definition: ctopology.h:254
int getLocalGateId() const
Definition: ctopology.h:264
cGate * getRemoteGate() const
Definition: ctopology.h:269
cGate * getLocalGate() const
Definition: ctopology.h:274
bool isEnabled() const
Definition: ctopology.h:115
int getModuleId() const
Definition: ctopology.h:92
Node * getLocalNode() const
Definition: ctopology.h:300
void setWeight(double d)
Definition: ctopology.h:109
A cObject that keeps track of its owner. It serves as base class for many classes in the OMNeT++ libr...
Definition: cownedobject.h:105