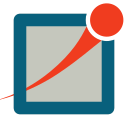 |
OMNeT++ API 6.2.0
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_CSOFTOWNER_H
17 #define __OMNETPP_CSOFTOWNER_H
19 #include "cownedobject.h"
44 #ifdef SIMFRONTEND_SUPPORT
46 int64_t lastChangeSerial;
51 virtual void ownedObjectDeleted(
cOwnedObject *obj)
override;
54 void reportUndisposed();
55 virtual void objectStealingOnDeletion(
cOwnedObject *obj);
61 #ifdef SIMFRONTEND_SUPPORT
63 void updateLastChangeSerial() {lastChangeSerial = changeCounter++;}
64 virtual bool hasChangedSince(int64_t lastRefreshSerial);
87 explicit cSoftOwner(
const char *name=
nullptr,
bool namepooling=
true);
106 virtual std::string str()
const override;
112 virtual void forEachChild(
cVisitor *v)
override;
cObject is a lightweight class which serves as the root of the OMNeT++ class hierarchy....
Definition: cobject.h:92
int getNumOwnedObjects() const
Definition: csoftowner.h:122
Enables traversing the tree of (cObject-rooted) simulation objects.
Definition: cvisitor.h:56
Internal class, used as a base class for modules and channels. It is not intended for subclassing out...
Definition: csoftowner.h:33
virtual bool isSoftOwner() const override
Definition: csoftowner.h:100
Abstract base class for creating a channel of a given type.
Definition: ccomponenttype.h:331
Base class for cOwnedObject-based classes that do not wish to support assignment and duplication.
Definition: cownedobject.h:242
A cObject that keeps track of its owner. It serves as base class for many classes in the OMNeT++ libr...
Definition: cownedobject.h:105