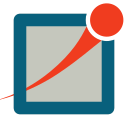 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_CRANDOM_H
17 #define __OMNETPP_CRANDOM_H
20 #include "cownedobject.h"
40 cRandom(
const char *name=
nullptr,
cRNG *rng=
nullptr);
62 virtual double draw()
const = 0;
86 cUniform(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double a=0,
double b=1) :
cRandom(name, rng), a(a), b(b) {}
90 virtual std::string str()
const override;
95 void setA(
double a) {this->a = a;}
96 double getA()
const {
return a;}
97 void setB(
double b) {this->b = b;}
98 double getB()
const {
return b;}
103 virtual double draw()
const override;
129 virtual std::string str()
const override;
134 void setMean(
double mean) {this->mean = mean;}
135 double getMean()
const {
return mean;}
140 virtual double draw()
const override;
159 void copy(
const cNormal& other);
163 cNormal(
cRNG *rng,
double mean,
double stddev) :
cRandom(rng), mean(mean), stddev(stddev) {}
164 cNormal(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double mean=0,
double stddev=1) :
cRandom(rng), mean(mean), stddev(stddev) {}
168 virtual std::string str()
const override;
173 void setMean(
double mean) {this->mean = mean;}
174 double getMean()
const {
return mean;}
175 void setStddev(
double stddev) {this->stddev = stddev;}
176 double getStddev()
const {
return stddev;}
181 virtual double draw()
const override;
205 cTruncNormal(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double mean=0,
double stddev=1) :
cRandom(rng), mean(mean), stddev(stddev) {}
209 virtual std::string str()
const override;
214 void setMean(
double mean) {this->mean = mean;}
215 double getMean()
const {
return mean;}
216 void setStddev(
double stddev) {this->stddev = stddev;}
217 double getStddev()
const {
return stddev;}
222 virtual double draw()
const override;
241 void copy(
const cGamma& other);
245 cGamma(
cRNG *rng,
double alpha,
double theta) :
cRandom(rng), alpha(alpha), theta(theta) {}
246 cGamma(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double alpha=1,
double theta=1) :
cRandom(rng), alpha(alpha), theta(theta) {}
250 virtual std::string str()
const override;
255 void setAlpha(
double alpha) {this->alpha = alpha;}
256 double getAlpha()
const {
return alpha;}
257 void setTheta(
double theta) {this->theta = theta;}
258 double getTheta()
const {
return theta;}
263 virtual double draw()
const override;
282 void copy(
const cBeta& other);
286 cBeta(
cRNG *rng,
double alpha1,
double alpha2) :
cRandom(rng), alpha1(alpha1), alpha2(alpha2) {}
287 cBeta(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double alpha1=1,
double alpha2=1) :
cRandom(rng), alpha1(alpha1), alpha2(alpha2) {}
291 virtual std::string str()
const override;
296 void setAlpha1(
double alpha1) {this->alpha1 = alpha1;}
297 double getAlpha1()
const {
return alpha1;}
298 void setAlpha2(
double alpha2) {this->alpha2 = alpha2;}
299 double getAlpha2()
const {
return alpha2;}
304 virtual double draw()
const override;
323 void copy(
const cErlang& other);
328 cErlang(
const char *name=
nullptr,
cRNG *rng=
nullptr,
unsigned int k=1,
double mean=1) :
cRandom(rng), k(k), mean(mean) {}
332 virtual std::string str()
const override;
337 void setK(
unsigned int k) {this->k = k;}
338 unsigned int getK()
const {
return k;}
339 void setMean(
double mean) {this->mean = mean;}
340 double getMean()
const {
return mean;}
345 virtual double draw()
const override;
372 virtual std::string str()
const override;
377 void setK(
unsigned int k) {this->k = k;}
378 unsigned int getK()
const {
return k;}
383 virtual double draw()
const override;
406 cStudentT(
const char *name=
nullptr,
cRNG *rng=
nullptr,
unsigned int i=1) :
cRandom(rng), i(i) {}
410 virtual std::string str()
const override;
415 void setI(
unsigned int i) {this->i = i;}
416 unsigned int getI()
const {
return i;}
421 virtual double draw()
const override;
440 void copy(
const cCauchy& other);
445 cCauchy(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double a=0,
double b=1) :
cRandom(rng), a(a), b(b) {}
449 virtual std::string str()
const override;
454 void setA(
double a) {this->a = a;}
455 double getA()
const {
return a;}
456 void setB(
double b) {this->b = b;}
457 double getB()
const {
return b;}
462 virtual double draw()
const override;
482 void copy(
const cTriang& other);
487 cTriang(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double a=-1,
double b=0,
double c=1) :
cRandom(rng), a(a), b(b), c(c) {}
491 virtual std::string str()
const override;
496 void setA(
double a) {this->a = a;}
497 double getA()
const {
return a;}
498 void setB(
double b) {this->b = b;}
499 double getB()
const {
return b;}
500 void setC(
double c) {this->c = c;}
501 double getC()
const {
return c;}
506 virtual double draw()
const override;
530 cWeibull(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double a=1,
double b=1) :
cRandom(rng), a(a), b(b) {}
534 virtual std::string str()
const override;
539 void setA(
double a) {this->a = a;}
540 double getA()
const {
return a;}
541 void setB(
double b) {this->b = b;}
542 double getB()
const {
return b;}
547 virtual double draw()
const override;
572 cParetoShifted(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double a=1,
double b=1,
double c=0) :
cRandom(rng), a(a), b(b), c(c) {}
576 virtual std::string str()
const override;
581 void setA(
double a) {this->a = a;}
582 double getA()
const {
return a;}
583 void setB(
double b) {this->b = b;}
584 double getB()
const {
return b;}
585 void setC(
double c) {this->c = c;}
586 double getC()
const {
return c;}
591 virtual double draw()
const override;
621 virtual std::string str()
const override;
626 void setA(
int a) {this->a = a;}
627 int getA()
const {
return a;}
628 void setB(
int b) {this->b = b;}
629 int getB()
const {
return b;}
634 virtual double draw()
const override;
662 virtual std::string str()
const override;
667 void setA(
int a) {this->a = a;}
668 int getA()
const {
return a;}
669 void setB(
int b) {this->b = b;}
670 int getB()
const {
return b;}
675 virtual double draw()
const override;
702 virtual std::string str()
const override;
707 void setP(
double p) {this->p = p;}
708 double getP()
const {
return p;}
713 virtual double draw()
const override;
737 cBinomial(
const char *name=
nullptr,
cRNG *rng=
nullptr,
int n=1,
double p=0.5) :
cRandom(rng), n(n), p(p) {}
741 virtual std::string str()
const override;
746 void setN(
int n) {this->n = n;}
747 int getN()
const {
return n;}
748 void setP(
double p) {this->p = p;}
749 double getP()
const {
return p;}
754 virtual double draw()
const override;
781 virtual std::string str()
const override;
786 void setP(
double p) {this->p = p;}
787 double getP()
const {
return p;}
792 virtual double draw()
const override;
820 virtual std::string str()
const override;
825 void setN(
int n) {this->n = n;}
826 int getN()
const {
return n;}
827 void setP(
double p) {this->p = p;}
828 double getP()
const {
return p;}
833 virtual double draw()
const override;
856 cPoisson(
const char *name=
nullptr,
cRNG *rng=
nullptr,
double lambda=1) :
cRandom(rng), lambda(lambda) {}
860 virtual std::string str()
const override;
865 void setLambda(
double lambda) {this->lambda = lambda;}
866 double getLambda()
const {
return lambda;}
871 virtual double draw()
const override;
virtual cParetoShifted * dup() const override
Definition: crandom.h:574
Generates random numbers from the truncated normal distribution.
Definition: crandom.h:194
Generates random numbers from the exponential distribution.
Definition: crandom.h:115
virtual cBinomial * dup() const override
Definition: crandom.h:739
virtual cGeometric * dup() const override
Definition: crandom.h:779
Generates random numbers from the beta distribution.
Definition: crandom.h:276
virtual cTruncNormal * dup() const override
Definition: crandom.h:207
virtual void setRNG(cRNG *rng)
Definition: crandom.h:49
virtual cNormal * dup() const override
Definition: crandom.h:166
Generates random numbers from the normal distribution.
Definition: crandom.h:153
Generates random numbers from the binomial distribution.
Definition: crandom.h:726
virtual cWeibull * dup() const override
Definition: crandom.h:532
virtual cBernoulli * dup() const override
Definition: crandom.h:700
Abstract interface for random variate generator classes.
Definition: crandom.h:31
Generates random numbers from the Cauchy distribution.
Definition: crandom.h:434
Generates random numbers from the chi-square distribution.
Definition: crandom.h:358
virtual cBeta * dup() const override
Definition: crandom.h:289
Generates random numbers that are the results of Bernoulli trials.
Definition: crandom.h:688
virtual cPoisson * dup() const override
Definition: crandom.h:858
Generates random numbers from Student's T distribution.
Definition: crandom.h:396
Generates random numbers from the Erlang distribution.
Definition: crandom.h:317
virtual cErlang * dup() const override
Definition: crandom.h:330
Abstract interface for random number generator classes.
Definition: crng.h:48
Generates random numbers from the Poisson distribution.
Definition: crandom.h:846
Generates random numbers from the shifted Pareto distribution.
Definition: crandom.h:560
Generates random numbers from the geometric distribution.
Definition: crandom.h:767
virtual cNegBinomial * dup() const override
Definition: crandom.h:818
Generates random numbers from the negative binomial distribution.
Definition: crandom.h:805
virtual cStudentT * dup() const override
Definition: crandom.h:408
virtual cExponential * dup() const override
Definition: crandom.h:127
virtual cGamma * dup() const override
Definition: crandom.h:248
virtual cChiSquare * dup() const override
Definition: crandom.h:370
virtual cTriang * dup() const override
Definition: crandom.h:489
virtual cCauchy * dup() const override
Definition: crandom.h:447
cRNG * getRNG() const
Definition: crandom.h:54
Generates random numbers from the Weibull distribution.
Definition: crandom.h:519
Generates random numbers from the gamma distribution.
Definition: crandom.h:235
A cObject that keeps track of its owner. It serves as base class for many classes in the OMNeT++ libr...
Definition: cownedobject.h:105
Generates random numbers from the triangular distribution.
Definition: crandom.h:475