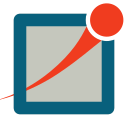 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_COUTVECTOR_H
17 #define __OMNETPP_COUTVECTOR_H
20 #include "cownedobject.h"
21 #include "simtime_t.h"
22 #include "opp_string.h"
67 void *handle =
nullptr;
74 void *dataForInspector;
78 void setCallback(
RecordFunc f,
void *d) {recordInInspector=f; dataForInspector=d;}
81 enum Type { TYPE_INT, TYPE_DOUBLE, TYPE_ENUM };
82 enum InterpolationMode { NONE, SAMPLE_HOLD, BACKWARD_SAMPLE_HOLD, LINEAR };
85 virtual void ensureRegistered();
115 virtual void setName(
const char *name)
override;
121 virtual std::string str()
const override;
137 virtual void setAttribute(
const char *name,
const char *value);
146 virtual void registerVector();
154 virtual void setEnum(
const char *registeredEnumName);
162 virtual void setEnum(
cEnum *enumDecl);
168 virtual void setUnit(
const char *unit);
175 virtual void setType(Type type);
182 virtual void setInterpolationMode(InterpolationMode mode);
189 virtual void setMin(
double minValue);
196 virtual void setMax(
double maxValue);
207 virtual bool record(
double value);
223 virtual bool recordWithTimestamp(
simtime_t t,
double value);
233 virtual void enable() {setFlag(FL_ENABLED,
true);}
239 virtual void disable() {setFlag(FL_ENABLED,
false);}
250 virtual bool isEnabled()
const {
return flags&FL_ENABLED;}
virtual void setEnabled(bool b)
Definition: coutvector.h:245
virtual void enable()
Definition: coutvector.h:233
Responsible for recording an "output vector" into the simulation results file. An output vector is a ...
Definition: coutvector.h:58
long getValuesReceived() const
Definition: coutvector.h:272
virtual bool getRecordDuringWarmupPeriod() const
Definition: coutvector.h:265
virtual bool isEnabled() const
Definition: coutvector.h:250
Lightweight string-to-string map, used internally in some parts of OMNeT++.
Definition: opp_string.h:219
long getValuesStored() const
Definition: coutvector.h:279
int64_t-based, base-10 fixed-point simulation time.
Definition: simtime.h:66
Provides string representation for enums.
Definition: cenum.h:33
SimTime simtime_t
Represents simulation time.
Definition: simtime_t.h:40
virtual bool recordWithTimestamp(simtime_t t, SimTime value)
Definition: coutvector.h:228
virtual bool record(SimTime value)
Definition: coutvector.h:212
double dbl() const
Definition: simtime.h:346
virtual void disable()
Definition: coutvector.h:239
void(* RecordFunc)(void *, simtime_t, double)
Prototype for callback functions that are used to notify graphical user interfaces when values are re...
Definition: coutvector.h:32
Base class for cOwnedObject-based classes that do not wish to support assignment and duplication.
Definition: cownedobject.h:242
virtual void setRecordDuringWarmupPeriod(bool b)
Definition: coutvector.h:259