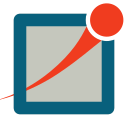 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_COBJECTFACTORY_H
17 #define __OMNETPP_COBJECTFACTORY_H
19 #include "simkerneldefs.h"
21 #include "cownedobject.h"
40 std::string description;
57 virtual std::string str()
const override;
66 virtual bool isAbstract()
const {
return creatorFunc==
nullptr;}
95 static cObjectFactory *find(
const char *className,
const char *contextNamespace=
nullptr,
bool fallbackToOmnetpp=
true);
100 static cObjectFactory *get(
const char *className,
const char *contextNamespace=
nullptr,
bool fallbackToOmnetpp=
true);
cObject * createOne(const char *classname)
Shortcut to cObjectFactory::createOne().
Definition: cobjectfactory.h:141
virtual bool isInstance(cObject *obj) const
Definition: cobjectfactory.h:80
virtual cObject * createOne() const
cObject is a lightweight class which serves as the root of the OMNeT++ class hierarchy....
Definition: cobject.h:92
The class behind the createOne() function and the Register_Class() macro.
Definition: cobjectfactory.h:35
cObject * createOneIfClassIsKnown(const char *classname)
Shortcut to cObjectFactory::createOneIfClassIsKnown().
Definition: cobjectfactory.h:149
virtual bool isAbstract() const
Definition: cobjectfactory.h:66
const char * getDescription() const
Definition: cobjectfactory.h:85
static cObject * createOneIfClassIsKnown(const char *classname)
Base class for cOwnedObject-based classes that do not wish to support assignment and duplication.
Definition: cownedobject.h:242