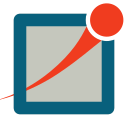 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_CARRAY_H
17 #define __OMNETPP_CARRAY_H
19 #include "cownedobject.h"
74 void init(
const cArray& a,
bool atHead=
true);
85 bool end()
const {
return k<0 || k>=array->
size();}
117 enum {FL_TKOWNERSHIP = 4};
118 cObject **vect =
nullptr;
125 void copy(
const cArray& other);
136 cArray(
const cArray& list);
142 explicit cArray(
const char *name=
nullptr,
int capacity=0,
int delta=10);
156 cArray& operator=(
const cArray& list);
173 virtual std::string str()
const override;
179 virtual void forEachChild(
cVisitor *v)
override;
186 virtual void parsimPack(
cCommBuffer *buffer)
const override;
193 virtual void parsimUnpack(
cCommBuffer *buffer)
override;
204 virtual int size()
const {
return last+1;}
210 virtual void clear();
221 virtual void setCapacity(
int capacity);
235 virtual int addAt(
int m,
cObject *obj);
251 virtual int find(
cObject *obj)
const;
258 virtual int find(
const char *objname)
const;
270 virtual cObject *get(
const char *objname);
276 virtual const cObject *get(
int m)
const;
282 virtual const cObject *get(
const char *objname)
const;
311 virtual bool exist(
int m)
const {
return m>=0 && m<=last && vect[m]!=
nullptr;}
317 virtual bool exist(
const char *objname)
const {
return find(objname)!=-1;}
324 virtual cObject *remove(
int m);
331 virtual cObject *remove(
const char *objname);
virtual int getCapacity() const
Definition: carray.h:215
virtual cObject * get(int m)
cObject is a lightweight class which serves as the root of the OMNeT++ class hierarchy....
Definition: cobject.h:92
Iterates through elements in a cArray, skipping holes (slots containing nullptr).
Definition: carray.h:53
Iterator & operator++()
Definition: carray.h:92
cObject * operator[](int m)
Definition: carray.h:288
const cObject * operator[](int m) const
Definition: carray.h:300
virtual cArray * dup() const override
Definition: carray.h:167
Iterator & operator--()
Definition: carray.h:106
Enables traversing the tree of (cObject-rooted) simulation objects.
Definition: cvisitor.h:56
Iterator operator++(int)
Definition: carray.h:99
Iterator operator--(int)
Definition: carray.h:113
cObject * operator*() const
Definition: carray.h:80
void setTakeOwnership(bool tk)
Definition: carray.h:362
Vector-like container for objects derived from cObject.
Definition: carray.h:38
bool end() const
Definition: carray.h:85
virtual int size() const
Definition: carray.h:204
cObject * operator[](const char *objname)
Definition: carray.h:294
virtual bool exist(const char *objname) const
Definition: carray.h:317
const cObject * operator[](const char *objname) const
Definition: carray.h:306
virtual bool exist(int m) const
Definition: carray.h:311
bool getTakeOwnership() const
Definition: carray.h:369
Iterator(const cArray &a, bool atHead=true)
Definition: carray.h:69
Buffer for the communications layer of parallel simulation.
Definition: ccommbuffer.h:41
A cObject that keeps track of its owner. It serves as base class for many classes in the OMNeT++ libr...
Definition: cownedobject.h:105