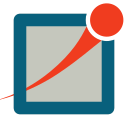 |
OMNeT++ API 6.1
Discrete Event Simulation Library
|
|
16 #ifndef __OMNETPP_CABSTRACTHISTOGRAM_H
17 #define __OMNETPP_CABSTRACTHISTOGRAM_H
39 Bin() {lower=upper=value=relativeFreq=0;}
40 std::string str()
const;
48 const Bin& internalGetBinInfo(
int k)
const;
88 virtual bool binsAlreadySetUp()
const = 0;
95 virtual void setUpBins() = 0;
101 virtual std::vector<double> getBinEdges()
const;
107 virtual std::vector<double> getBinValues()
const;
112 virtual int getNumBins()
const = 0;
121 virtual double getBinEdge(
int k)
const = 0;
127 virtual double getBinValue(
int k)
const = 0;
134 virtual double getBinPDF(
int k)
const;
141 virtual int64_t getNumUnderflows()
const = 0;
148 virtual int64_t getNumOverflows()
const = 0;
155 virtual double getUnderflowSumWeights()
const = 0;
162 virtual double getOverflowSumWeights()
const = 0;
167 virtual int64_t getNumNegInfs()
const = 0;
172 virtual int64_t getNumPosInfs()
const = 0;
177 virtual double getNegInfSumWeights()
const = 0;
182 virtual double getPosInfSumWeights()
const = 0;
188 virtual Bin getBinInfo(
int k)
const;
197 virtual double getPDF(
double x)
const;
202 virtual double getCDF(
double x)
const;
207 virtual double draw()
const override;
cAbstractHistogram & operator=(const cAbstractHistogram &res)
Definition: cabstracthistogram.h:72
Information about a histogram bin. This struct is not used internally by the histogram classes,...
Definition: cabstracthistogram.h:33
cAbstractHistogram(const char *name=nullptr, bool weighted=false)
Definition: cabstracthistogram.h:62
virtual ~cAbstractHistogram()
Definition: cabstracthistogram.h:67
virtual cAbstractHistogram * dup() const override
Definition: cabstracthistogram.h:80
Interface and base class for histogram-like density estimation classes.
Definition: cabstracthistogram.h:26
Statistics class to collect min, max, mean, and standard deviation.
Definition: cstddev.h:29
Thrown when the simulation kernel or other components detect a runtime error.
Definition: cexception.h:286
cStdDev & operator=(const cStdDev &res)